How to Automate UI Testing: A Step-by-Step Guide
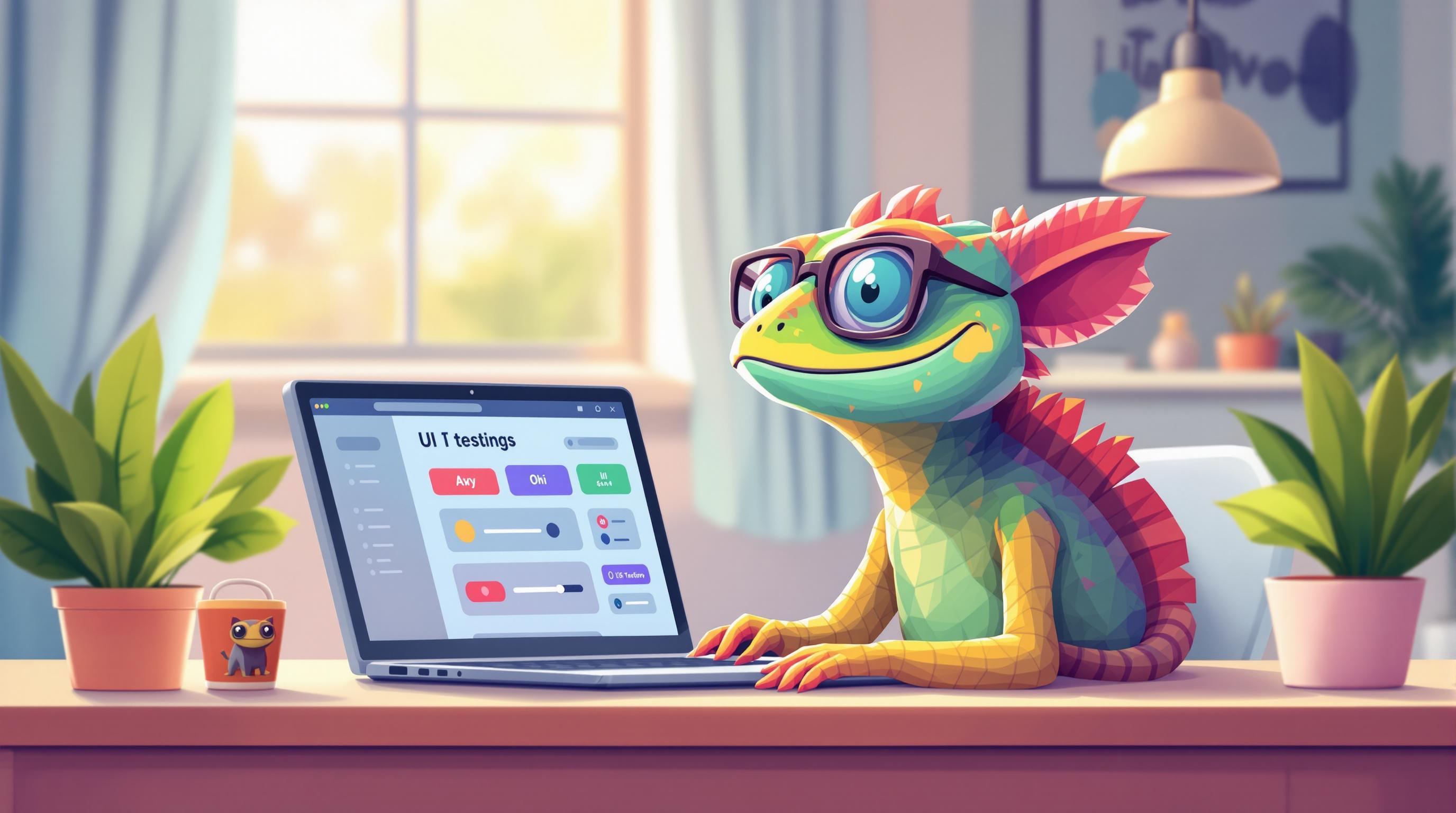
How to Automate UI Testing: A Step-by-Step Guide
Automating UI testing can save up to 80% of testing time and reduce post-release defects by 40%. It’s essential for faster release cycles and better software quality. Here's what you need to know:
- Why Automate? Speeds up testing, improves quality, and ensures fewer defects.
- Best Tools: Top picks include Selenium, Cypress, and Playwright for web, and Appium for mobile.
- Setup Tips: Use Docker for consistent environments, generate test data programmatically, and integrate with cloud testing platforms.
- Best Practices: Use the Page Object Model (POM), stable locators, and retry logic for reliable scripts.
- CI/CD Integration: Automate tests in pipelines with tools like GitHub Actions, enabling parallel execution and real-time reporting.
Quick Comparison of Tools
Tool | Key Features | Ideal For | Supported Languages |
---|---|---|---|
Selenium | Cross-browser, large ecosystem | Large-scale projects | Java, Python, C#, Ruby, JS |
Cypress | Real-time debugging, auto-waiting | Modern web apps | JavaScript |
Playwright | Cross-platform, fast execution | Multi-browser testing | JS, TypeScript, Python, .NET |
Start with critical workflows, pick the right tools, and integrate testing into your CI/CD pipeline for faster, more reliable results.
Selenium Vs Cypress Vs Playwright: Key Differences at a Glance
Selecting UI Automation Tools
Picking the right UI automation tool can greatly influence both testing efficiency and long-term costs. When it comes to web testing, there are three standout options, each offering its own set of advantages:
Top UI Testing Tools Compared
Tool | Key Features | Ideal For | Supported Languages |
---|---|---|---|
Selenium | Cross-browser testing, extensive ecosystem | Large-scale projects | Java, Python, C#, Ruby, JavaScript |
Cypress | Real-time debugging, automatic waiting | Modern web apps | JavaScript |
Playwright | Cross-platform, built-in auto-waiting | Multi-browser testing | JavaScript, TypeScript, Python, .NET, Java |
According to third-party benchmarks, Playwright excels in cross-browser automation due to its highly efficient browser control capabilities [3].
Tools for Specific Testing Needs
For mobile testing, Appium stands out with its cross-platform capabilities. If you're looking for AI-driven solutions, TestCraft uses AI to handle UI changes by recognizing elements dynamically.
When evaluating tools, focus on these key aspects:
-
Integration Requirements
Ensure the tool integrates well with your CI/CD pipeline. For instance, Playwright's built-in test runner pairs seamlessly with modern JavaScript frameworks, while Selenium's ecosystem supports a wide range of testing frameworks. -
Maintenance Overhead
Opt for tools that minimize flaky tests. Cypress, for example, automatically waits for elements and retries failed tests, reducing maintenance efforts significantly. -
Execution Speed
Tools like Playwright leverage efficient browser automation, offering faster test execution compared to older solutions.
Once you've chosen a tool that fits your needs, the next step is to configure your testing environment for optimal performance.
Test Environment Setup
To ensure effective testing, create an environment that mirrors production while allowing for quick testing cycles. A reliable test environment is key to consistent automation. Start by outlining test coverage with a matrix prioritizing user journeys:
Planning Test Coverage
Test Type | Priority | Coverage Areas |
---|---|---|
Critical Flows | High | Login, Checkout, Core Features |
Visual Elements | Medium | Layout, Responsiveness |
Edge Cases | Medium | Error States, Boundary Values |
Accessibility | High | WCAG Compliance |
Focus first on business-critical paths. For example, e-commerce platforms should automate checkout processes before tackling secondary features.
Environment Configuration Steps
Here are the essential steps to set up your test environment:
-
Container Setup
Use a base Docker configuration to standardize the environment:
Containerization ensures consistency across environments, reducing testing time by up to 80% [2].FROM ubuntu:20.04 RUN apt-get update && apt-get install -y \ openjdk-11-jdk \ firefox \ xvfb RUN wget https://github.com/mozilla/geckodriver/releases/download/v0.30.0/geckodriver-v0.30.0-linux64.tar.gz \ && tar -xzf geckodriver-v0.30.0-linux64.tar.gz \ && mv geckodriver /usr/local/bin/
-
Data Management
Programmatically generate test data to cover various scenarios:
Synthetic data improves scenario coverage, contributing to a 40% reduction in defects [2].from faker import Faker fake = Faker() def generate_test_user(): return { "name": fake.name(), "email": fake.email(), "address": fake.address() }
-
Cloud Integration
Set up cloud-based device testing for broader compatibility:
This setup lays the groundwork for creating efficient scripts, a key step in UI automation.desired_cap = { 'browserName': 'iPhone', 'device': 'iPhone 11', 'realMobile': 'true', 'os_version': '14.0' }
sbb-itb-b77241c
Creating and Managing Test Scripts
Writing effective UI test scripts requires a focus on making them easy to maintain and ensuring they remain stable over time. This can be achieved through a mix of smart structuring, AI-driven tools, and robust error management.
Test Script Writing Guidelines
The Page Object Model (POM) is a go-to approach for organizing UI tests. It separates the test logic from UI element definitions, making your scripts easier to manage and update. Here's an example of POM in action using Playwright:
from playwright.sync_api import Page
class ProductPage:
def __init__(self, page: Page):
self.page = page
self.add_to_cart = page.locator("[data-testid='add-to-cart']")
self.quantity = page.locator("#quantity-input")
self.cart_count = page.locator(".cart-badge")
def add_product(self, quantity: int):
self.quantity.fill(str(quantity))
self.add_to_cart.click()
return self.cart_count.text_content()
To make your test scripts more reliable, follow these best practices:
Practice | How to Use It | Benefits |
---|---|---|
Explicit Waits | Use waitForSelector() instead of hard-coded delays |
Reduces flaky tests |
Stable Locators | Rely on data-testid attributes |
Lowers maintenance effort |
Error Handling | Add retry mechanisms | Boosts reliability |
Using AI for Test Updates
AI tools are changing the game for test maintenance. They offer features like automatic test healing and smarter ways to locate elements. Here's a quick comparison:
# Traditional method requiring constant updates
button = driver.find_element(By.ID, "submit-button")
# AI-powered method that adapts to UI changes
button = AI_powered_locator("Submit Order", context="checkout-flow")
By leveraging AI, you can reduce the time spent on manually updating locators every time the UI changes.
Fixing Common Test Problems
Stability issues in tests often stem from challenges like dynamic content or timing mismatches. Here's how to tackle them:
- Retry Logic for Dynamic Elements: Use retry mechanisms to handle elements that may not be immediately available.
@retry(max_attempts=3)
def click_dynamic_element():
element.click()
- Parallel Execution: For performance bottlenecks, run tests in parallel through your CI/CD pipeline.
Problem | Solution |
---|---|
Dynamic Content | Combine retry logic with explicit waits |
Performance Issues | Enable parallel execution in CI/CD |
Adding UI Tests to CI/CD
Once you've built reliable test scripts, the next step is to integrate them into your CI/CD pipeline for continuous validation. Tools like GitHub Actions make this process straightforward.
CI/CD Test Setup Guide
Here's an example configuration for running UI tests in a CI/CD pipeline:
name: UI Tests
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
container:
image: mcr.microsoft.com/playwright:v1.40.0
steps:
- uses: actions/checkout@v3
- name: Install dependencies
run: npm ci
- name: Run UI tests
run: npx playwright test
- name: Store test results
if: always()
uses: actions/upload-artifact@v3
with:
name: playwright-report
path: playwright-report/
Speeding Up Test Execution
To make your tests run faster, you can use parallel execution with matrix strategies. Here's an example:
jobs:
test:
runs-on: ubuntu-latest
strategy:
matrix:
shard: [1, 2, 3, 4]
steps:
- uses: actions/checkout@v3
- name: Run tests
run: npx playwright test --shard=${{ matrix.shard }}/4
Additionally, you can optimize further by executing only the tests impacted by recent changes, using changed-file filters.
Test Results and Reports
Turn test results into actionable insights with tools like Allure for interactive reporting:
- name: Generate Allure Report
uses: simple-elf/allure-report-action@master
if: always()
with:
allure_results: allure-results
gh_pages: gh-pages
allure_report: allure-report
Organizations that adopt automated UI testing within their CI/CD workflows have seen up to 60% fewer defects after release[1]. For even better results, consider adding tools like Percy to validate visual layouts.
To get the most out of your testing setup:
- Set up automatic notifications for test failures to stay informed.
- Track test flakiness and stability trends to identify and resolve recurring issues.
- Enable retry mechanisms to handle intermittent failures:
# playwright.config.ts
export default { retries: 3 }
Conclusion
Once you've implemented CI/CD-integrated tests, the next step is maintaining automation efficiency with smart, ongoing updates.
Key Takeaways
Modern tools like Playwright have made UI test automation three times faster[3]. By using a priority matrix during setup, you can focus on the areas that matter most, which boosts returns significantly. This approach has led to a 40% drop in post-release defects and an 80% reduction in testing time[2].
Steps to Begin
Follow these four phases to kick off UI automation:
- Review and prioritize: Audit your current tests and focus on the flows that have the biggest impact.
- Use the right tools: Set up tools that match your environment for smoother integration.
- Integrate with CI/CD: Use parallel execution to streamline processes.
- Keep it fresh: Track flaky tests and refresh locators weekly to maintain reliability.
This phased approach builds on CI/CD integration, ensuring a constant flow of quality feedback.
FAQs
How to integrate automated tests into a CI/CD pipeline?
To incorporate automated tests into your CI/CD pipeline effectively, follow these steps:
- Set Up Test Tools: Connect your testing framework (like Jenkins, GitLab, or Azure DevOps) with your CI/CD platform. Make sure the tools work smoothly within your existing workflow.
- Prepare Test Environments: Create testing environments that closely resemble your production setup. Keep these environments isolated to ensure accurate results and maintain data integrity.
-
Streamline Test Execution: Focus on running essential tests first and use parallel execution to speed things up. You can also optimize by:
- Prioritizing tests critical to the application.
- Running only tests impacted by recent code changes.
- Using dedicated test accounts to maintain consistency in data.